Note
Go to the end to download the full example code.
Custom plots in 2D¶
Create a new 2D plot based on output data.
Download Input files
from roxieapi.commons.roxie_constants import PlotLabels
from roxieapi.commons.types import Plot2D
from roxieapi.output.plots import RoxiePlotOutputs
Setting up¶
Standard Loading of input, Parsing and Initializing RoxiePlotOutputs object
plots = RoxiePlotOutputs(
"../input_files/dipole_iron.post.xml", "../input_files/dipole_iron.data"
)
Plots defined in Roxie¶
Print a list of Plots which are defined in Roxie
for pl in PlotLabels.plot2D_desc:
lbl, desc = PlotLabels.lbl_desc_plot2D(pl)
print(f"Coil plot {pl}, Label: '{lbl}', Description: '{desc}'")
for pl in PlotLabels.plotMesh2D_desc:
lbl, desc = PlotLabels.lbl_desc_mesh2D(pl)
print(f"Mesh plot {pl}, Label: '{lbl}', Description: '{desc}'")
Coil plot 1, Label: 'BX', Description: 'Bx (T)'
Coil plot 2, Label: 'BY', Description: 'By (T)'
Coil plot 3, Label: '|B|', Description: '|B| (T)'
Coil plot 4, Label: 'B', Description: 'Magnetic flux density (T)'
Coil plot 5, Label: 'BPERP', Description: 'B normal to broad side (T) r x Bperp positive in z'
Coil plot 6, Label: 'BPARA', Description: 'B tangent. to broad side (T) pos. in outward direction'
Coil plot 7, Label: 'FX', Description: 'Fx / L (N/M)'
Coil plot 8, Label: 'FY', Description: 'Fy / L (N/M)'
Coil plot 9, Label: '|F|', Description: '|F| / L (N/M)'
Coil plot 10, Label: 'F', Description: 'Emag. force / L (N/m)'
Coil plot 11, Label: 'FPERP', Description: 'F normal to broad side / L (N/M)'
Coil plot 12, Label: 'FPARA', Description: 'F tangential broad side / L (N/M)pos. in outward direction'
Coil plot 13, Label: 'A', Description: 'Vector potential (Tm)'
Coil plot 14, Label: 'I', Description: 'I (A)'
Coil plot 15, Label: 'JELE', Description: 'J (A/mm^2!)'
Coil plot 16, Label: 'JCU', Description: 'J-Cu (A/mm^2!)'
Coil plot 17, Label: 'JSC', Description: 'J-Sc (A/mm^2!)'
Coil plot 18, Label: 'MARG', Description: 'Margin to quench (%)'
Coil plot 19, Label: 'BREDX', Description: 'Bred x-comp (T)'
Coil plot 20, Label: 'BREDY', Description: 'Bred y-comp (T)'
Coil plot 21, Label: 'BR', Description: '|Bred| (T)'
Coil plot 22, Label: 'ARED', Description: 'Ared (Tm)'
Coil plot 24, Label: '|I|', Description: '|I| (A)'
Coil plot 25, Label: '|JEL|', Description: '|J| (A/mm^2!)'
Coil plot 26, Label: '|JCU|', Description: '|J-Cu| (A/mm^2!)'
Coil plot 27, Label: '|JSC|', Description: '|J-Sc| (A/mm^2!)'
Coil plot 28, Label: 'MARGT', Description: 'Temperature margin (K)'
Coil plot 29, Label: 'T', Description: 'T (K)'
Coil plot 36, Label: 'MQES', Description: 'Enthalpy Margin Strand (mJ/cm^3!)'
Coil plot 37, Label: 'MQEC1', Description: 'Enthalpy Margin Cable 1 (mJ/cm^3!)'
Coil plot 38, Label: 'MQEC2', Description: 'Enthalpy Margin Cable 2 (mJ/cm^3!)'
Coil plot 39, Label: 'PINT', Description: 'P integrated (Ws/m)'
Coil plot 40, Label: 'MPHAS', Description: 'Alpha M (deg)'
Coil plot 41, Label: 'MX', Description: 'Mx (A/m)'
Coil plot 42, Label: 'MY', Description: 'My (A/m)'
Coil plot 43, Label: '|M|', Description: 'M (A/m)'
Coil plot 44, Label: 'M', Description: 'SC filament magn. (A/m)'
Coil plot 45, Label: 'FPN', Description: 'F || / F -|'
Coil plot 46, Label: 'MMOD', Description: '|M| (A/m)'
Coil plot 47, Label: 'BPHAS', Description: 'Alpha B (deg)'
Coil plot 48, Label: 'P', Description: 'P (W/m)'
Coil plot 49, Label: 'B1', Description: 'B1 Contrib. of I strand (T)'
Coil plot 50, Label: 'B2', Description: 'B2 Contrib. of I strand (T)'
Coil plot 51, Label: 'B3', Description: 'B3 Contrib. of I strand (T)'
Coil plot 52, Label: 'B4', Description: 'B4 Contrib. of I strand (T)'
Coil plot 53, Label: 'B5', Description: 'B5 Contrib. of I strand (T)'
Coil plot 54, Label: 'B6', Description: 'B6 Contrib. of I strand (T)'
Coil plot 55, Label: 'B7', Description: 'B7 Contrib. of I strand (T)'
Coil plot 56, Label: 'B8', Description: 'B8 Contrib. of I strand (T)'
Coil plot 57, Label: 'B9', Description: 'B9 Contrib. of I strand (T)'
Coil plot 58, Label: 'B10', Description: 'B10 Contrib. of I strand (T)'
Coil plot 59, Label: 'B11', Description: 'B11 Contrib. of I strand (T)'
Coil plot 60, Label: 'M1', Description: 'B1 Contrib. of M strand (T)'
Coil plot 61, Label: 'M2', Description: 'B2 Contrib. of M strand (T)'
Coil plot 62, Label: 'M3', Description: 'B3 Contrib. of M strand (T)'
Coil plot 63, Label: 'M4', Description: 'B4 Contrib. of M strand (T)'
Coil plot 64, Label: 'M5', Description: 'B5 Contrib. of M strand (T)'
Coil plot 65, Label: 'M6', Description: 'B6 Contrib. of M strand (T)'
Coil plot 66, Label: 'M7', Description: 'B7 Contrib. of M strand (T)'
Coil plot 67, Label: 'M8', Description: 'B8 Contrib. of M strand (T)'
Coil plot 68, Label: 'M9', Description: 'B9 Contrib. of M strand (T)'
Coil plot 69, Label: 'M10', Description: 'B10 Contrib. of M strand (T)'
Coil plot 70, Label: 'M11', Description: 'B11 Contrib. of M strand (T)'
Coil plot 79, Label: 'B1ICC', Description: 'B1 Contrib. of ICC (T)'
Coil plot 80, Label: 'B2ICC', Description: 'B2 Contrib. of ICC (T)'
Coil plot 81, Label: 'B3ICC', Description: 'B3 Contrib. of ICC (T)'
Coil plot 82, Label: 'B4ICC', Description: 'B4 Contrib. of ICC (T)'
Coil plot 83, Label: 'B5ICC', Description: 'B5 Contrib. of ICC (T)'
Coil plot 84, Label: 'B6ICC', Description: 'B6 Contrib. of ICC (T)'
Coil plot 85, Label: 'B7ICC', Description: 'B7 Contrib. of ICC (T)'
Coil plot 86, Label: 'B8ICC', Description: 'B8 Contrib. of ICC (T)'
Coil plot 87, Label: 'B9ICC', Description: 'B9 Contrib. of ICC (T)'
Coil plot 88, Label: 'B10ICC', Description: 'B10 Contrib. of ICC (T)'
Coil plot 89, Label: 'B11ICC', Description: 'B11 Contrib. of ICC (T)'
Coil plot 91, Label: 'ICC', Description: 'I-CC (A)'
Coil plot 92, Label: 'A1ICC', Description: 'A1 Contrib. of ICC (T)'
Coil plot 93, Label: 'A2ICC', Description: 'A2 Contrib. of ICC (T)'
Coil plot 94, Label: 'A3ICC', Description: 'A3 Contrib. of ICC (T)'
Coil plot 95, Label: 'A4ICC', Description: 'A4 Contrib. of ICC (T)'
Coil plot 96, Label: 'A5ICC', Description: 'A5 Contrib. of ICC (T)'
Coil plot 97, Label: 'A6ICC', Description: 'A6 Contrib. of ICC (T)'
Coil plot 146, Label: 'B12', Description: 'B12 Contrib. of I strand (T)'
Coil plot 147, Label: 'B13', Description: 'B13 Contrib. of I strand (T)'
Coil plot 148, Label: 'B14', Description: 'B14 Contrib. of I strand (T)'
Coil plot 155, Label: 'V', Description: 'Voltage to Clamp (V)'
Coil plot 161, Label: 'A1', Description: 'A1 Contrib. of I strand (T)'
Coil plot 162, Label: 'A2', Description: 'A2 Contrib. of I strand (T)'
Coil plot 163, Label: 'A3', Description: 'A3 Contrib. of I strand (T)'
Coil plot 164, Label: 'A4', Description: 'A4 Contrib. of I strand (T)'
Coil plot 165, Label: 'A5', Description: 'A5 Contrib. of I strand (T)'
Coil plot 166, Label: 'A6', Description: 'A6 Contrib. of I strand (T)'
Coil plot 167, Label: 'A7', Description: 'A7 Contrib. of I strand (T)'
Coil plot 168, Label: 'A8', Description: 'A8 Contrib. of I strand (T)'
Coil plot 169, Label: 'A9', Description: 'A9 Contrib. of I strand (T)'
Coil plot 170, Label: 'A10', Description: 'A10 Contrib. of I strand (T)'
Coil plot 171, Label: 'A11', Description: 'A11 Contrib. of I strand (T)'
Coil plot 172, Label: 'A12', Description: 'A12 Contrib. of I strand (T)'
Coil plot 173, Label: 'A13', Description: 'A13 Contrib. of I strand (T)'
Coil plot 174, Label: 'A14', Description: 'A14 Contrib. of I strand (T)'
Coil plot 360, Label: 'MARGJ', Description: 'Current density margin(at Jop,Bop,Top)(A/mm2)'
Mesh plot 31, Label: 'MUER', Description: 'Muer'
Mesh plot 32, Label: '|BTOT|', Description: '|B| (T)'
Mesh plot 34, Label: 'AR', Description: 'Az (Tm)'
Mesh plot 35, Label: 'MUEFAC', Description: '(Muer-1)/(Muer+1)'
Mesh plot 75, Label: 'Bx', Description: 'Bx (T)'
Mesh plot 76, Label: 'By', Description: 'By (T)'
Plots defined in Roxie¶
Print all plots available of an output
trans = plots.output.opt[1].step[1]
print("Coil Plots:")
data_cols = trans.coilData.columns
for col in data_cols:
if col in PlotLabels.plot2D_desc:
lbl, desc = PlotLabels.lbl_desc_plot2D(col)
print(f" Plot {col}: {desc}")
for hc_idx, hc in trans.harmonicCoils.items():
data_cols = hc.strandData
for col in data_cols:
if col in PlotLabels.plot2D_desc:
lbl, desc = PlotLabels.lbl_desc_plot2D(col)
print(f" Harmonic coil #{hc_idx}, Plot {col}: {desc}")
print("Iron mesh plots:")
data_cols = plots.output.opt[1].step[1].meshData.columns
for col in data_cols:
if col in PlotLabels.plotMesh2D_desc:
lbl, desc = PlotLabels.lbl_desc_mesh2D(col)
print(f" Plot {col}: {desc}")
Coil Plots:
Plot 14: I (A)
Plot 15: J (A/mm^2!)
Plot 16: J-Cu (A/mm^2!)
Plot 17: J-Sc (A/mm^2!)
Plot 24: |I| (A)
Plot 25: |J| (A/mm^2!)
Plot 26: |J-Cu| (A/mm^2!)
Plot 27: |J-Sc| (A/mm^2!)
Plot 29: T (K)
Plot 1: Bx (T)
Plot 2: By (T)
Plot 3: |B| (T)
Plot 4: Magnetic flux density (T)
Plot 5: B normal to broad side (T) r x Bperp positive in z
Plot 6: B tangent. to broad side (T) pos. in outward direction
Plot 7: Fx / L (N/M)
Plot 8: Fy / L (N/M)
Plot 9: |F| / L (N/M)
Plot 10: Emag. force / L (N/m)
Plot 11: F normal to broad side / L (N/M)
Plot 12: F tangential broad side / L (N/M)pos. in outward direction
Plot 13: Vector potential (Tm)
Plot 45: F || / F -|
Plot 47: Alpha B (deg)
Plot 360: Current density margin(at Jop,Bop,Top)(A/mm2)
Plot 19: Bred x-comp (T)
Plot 20: Bred y-comp (T)
Plot 21: |Bred| (T)
Plot 22: Ared (Tm)
Harmonic coil #1, Plot 49: B1 Contrib. of I strand (T)
Harmonic coil #1, Plot 50: B2 Contrib. of I strand (T)
Harmonic coil #1, Plot 51: B3 Contrib. of I strand (T)
Harmonic coil #1, Plot 52: B4 Contrib. of I strand (T)
Harmonic coil #1, Plot 53: B5 Contrib. of I strand (T)
Harmonic coil #1, Plot 54: B6 Contrib. of I strand (T)
Harmonic coil #1, Plot 55: B7 Contrib. of I strand (T)
Harmonic coil #1, Plot 56: B8 Contrib. of I strand (T)
Harmonic coil #1, Plot 57: B9 Contrib. of I strand (T)
Harmonic coil #1, Plot 58: B10 Contrib. of I strand (T)
Harmonic coil #1, Plot 59: B11 Contrib. of I strand (T)
Harmonic coil #1, Plot 146: B12 Contrib. of I strand (T)
Harmonic coil #1, Plot 147: B13 Contrib. of I strand (T)
Harmonic coil #1, Plot 148: B14 Contrib. of I strand (T)
Harmonic coil #1, Plot 161: A1 Contrib. of I strand (T)
Harmonic coil #1, Plot 162: A2 Contrib. of I strand (T)
Harmonic coil #1, Plot 163: A3 Contrib. of I strand (T)
Harmonic coil #1, Plot 164: A4 Contrib. of I strand (T)
Harmonic coil #1, Plot 165: A5 Contrib. of I strand (T)
Harmonic coil #1, Plot 166: A6 Contrib. of I strand (T)
Harmonic coil #1, Plot 167: A7 Contrib. of I strand (T)
Harmonic coil #1, Plot 168: A8 Contrib. of I strand (T)
Harmonic coil #1, Plot 169: A9 Contrib. of I strand (T)
Harmonic coil #1, Plot 170: A10 Contrib. of I strand (T)
Harmonic coil #1, Plot 171: A11 Contrib. of I strand (T)
Harmonic coil #1, Plot 172: A12 Contrib. of I strand (T)
Harmonic coil #1, Plot 173: A13 Contrib. of I strand (T)
Harmonic coil #1, Plot 174: A14 Contrib. of I strand (T)
Iron mesh plots:
Plot 31: Muer
Plot 32: |B| (T)
Plot 34: Az (Tm)
Plot 35: (Muer-1)/(Muer+1)
Plot 75: Bx (T)
Plot 76: By (T)
Define a new Plot and create it¶
From the list of available plots, Select which fields to plot, and create the output
plot_created = Plot2D.create("My Plot2D")
plot_created.add_coilPlot("52", harm_coil=1)
# pl.add_meshPlot("35", legend=PlotLegend("e", False, 0, 1))
figure = plots.plots2d.plot_xs(plot_created)
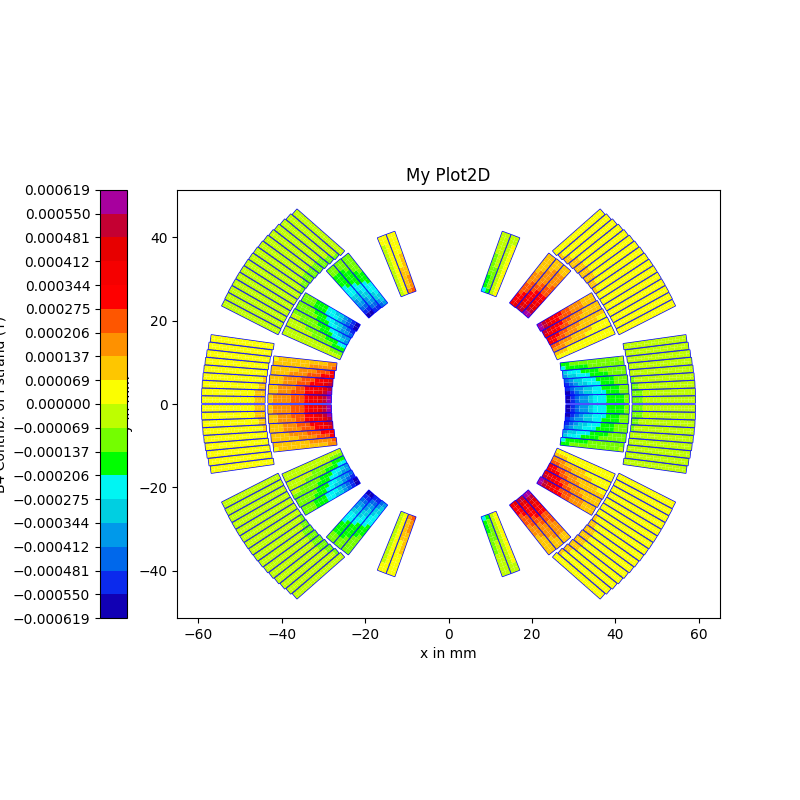
Total running time of the script: (0 minutes 12.116 seconds)